Computer Science 15-100 (Sections S-V), Fall 2008
Homework 6
Due: Fri 10-Oct-2008 at 7:59pm
(email copy) and at your next
class/recitation
(identical physical copy)
(no late submissions accepted).
Read these instructions first!
- Be sure to include your name, your Andrew ID, and your section clearly on the top of each file in your assignment.
- Also on the top of each file, include a timesheet logging all
the time you spent on that part of the assignment.
You will not be graded on your number of hours, but this information
will be helpful to the course staff.
- For non-programming problems (there are none)
- Place all your solutions to the non-programming
problems in a single file named Hw6 (with whatever extension is appropriate
for the format you choose, such as Hw6.txt or Hw6.html, etc). You must
use a one of these file formats: plain text (txt), or RTF, or HTML, or
Word (doc, not docx), or PDF. No other file formats will be accepted.
- Show your work. Correct
answers without supporting calculations will not receive full credit.
- For programming problems:
- Place each solution in its own file named exactly as given below, and
with a class name that exactly matches the file name. So if the file
name is Hw6Foo.java, the main class in that file must be Hw6Foo.
- Use well-named variables, proper indenting, reasonable commenting,
etc.
- What to submit
- Either one zip file, Hw6.zip, containing all your files (this is
preferred), or all the files as attachments to a single email (do not send
one email per file!). It is recommended
that you "cc" yourself in that email, too, just to confirm that you properly
sent the email.
- ArrayPractice
- scrabbleScore
- wordsInWord
- isMagicSquare
- Connect4
-
Bonus/Optional:
Kepler's Polygons
-
Bonus/Optional: Othello/Reversi
- Array Practice
In a file named Hw6ArrayPractice.java, write each of the following methods
along with their corresponding well-designed and aptly-named test methods.
- scrabbleScore
In the game of Scrabble, players receive points for spelling words,
where each letter has an associated point value (1 for A, 3 for B, 3 for C,
2 for D, and so on...). For example, ignoring special squares (which
we will), the word "CAB" is worth 3+1+3, or 7 points. For more
details, here is the Wikipedia page on Scrabble:
http://en.wikipedia.org/wiki/Scrabble
Write the following method:
public
static int scrabbleScore(String s, int[] letterValues)
This method takes a String s and an array of 26 integers representing point
values of the letters from A to Z in order, and returns the sum of the point
values for each letter in the string. If the string is null or empty
or if it contains any characters besides uppercase letters, or if the
letterValues array is null or not of length 26, the result should be -1 to
indicate an error.
If you are interested, here is an array holding the actual letter values for
the English-language version of Scrabble:
private
static int[] letterValues = {
// A, B, C, D, E, F, G, H, I, J, K, L, M
1, 3, 3, 2, 1, 4, 2, 4, 1, 8, 5, 1, 3,
// N, O, P, Q, R, S, T, U, V, W, X, Y, Z
1, 1, 3,10, 1, 1, 1, 1, 4, 4, 8, 4,10
};
Note: The letterValues array may contain point values besides
the official point values (say, 25 for 'A', 18 for 'B', -33 for 'C', and so
on). Your method must work for whatever letterValues array is
provided.
- wordsInWord
Write the following method:
public
static String[] wordsInWord(String s, String[] dictionary)
This method takes a string s and a possibly-unsorted array of ALL-CAPS strings, the
dictionary, and returns a sorted array containing all the substrings of s
(excluding s itself) that appear (case-insensitively) in the dictionary. So, for example,
given the word "abcd" and the dictionary { "D", "ABCD", "AC", "BD", "BC"},
your method would return the array { "BC", "D" }. If either the string
s or the dictionary array is null, your method should return null.
However, if both are non-null and there are no substrings in the dictionary,
your method should return a non-null array of size zero. Also, no word
should appear more than once in the returned array, even if that word occurs
more than once as a substring and is in the dictionary.
- isMagicSquare
According to the
Mathworld page on Magic Squares, a magic square is "a square
array of numbers consisting of the distinct positive integers 1, 2, ..., n2
arranged such that the sum of the n numbers in any horizontal, vertical, or
main diagonal line is always the same number." For example, here is a
magic square:
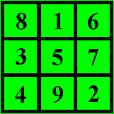
This square is magic because in contains the numbers 1, 2, ..., 9, and
all the rows, columns, and diagonals sum to the same number (15).
Write the following method:
public
static boolean isMagicSquare(int[][] a)
This method takes a 2d-array of int's and returns true if it represents a
magic square, and false otherwise. Note that non-square matrices
and null matrices are not magic squares, so your method should return false
in these cases.
Note: magic squares do not have to be 3x3. They can be as
small as 1x1 or arbitrarily larger.
- Connect4
In a file named
Hw6Connect4.java, write the game of Connect4:
connect4.html (applet)
or connect4.jar (app)
To play the game: Two players alternate turns dropping pieces (using
the left, right, and down or space keys) in columns. First player to
get 4-in-a-row wins that game. If the board fills up, that game is a
tie. Play the game a bit to understand the rest of the design spec.
The game IS the design spec, so your games should match its behavior (though
you do not have to be "pixel perfect" -- just "very close"). For more
details, here is the Wikipedia page on Connect4:
http://en.wikipedia.org/wiki/Connect_Four
-
Bonus/Optional:
Kepler's Polygons
Note: This is a repeat from last week, as most of you did not
take advantage of this really interesting bonus problem. This week,
you may again do up to any two of these but you may not repeat any that you
submitted last week.
Among his lesser-known exploits, the great German mathematician and
astronomer Johannes Kepler studied how polygons can tile the plan or
portions of the plane. As part of these studies, he created these
interesting figures:
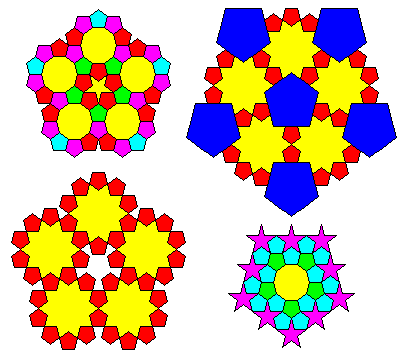
In a file named Hw6BonusKeplerPolygon.java, paint any one or two of these
four figures.
Note that the lower-left figure is worth half as many bonus points as the
other three figures. For this problem only, you do not have to make
the figures scale according to the size of the window, but you do have to
use loops as much as possible (which is an interesting conundrum) rather
than manually place each polygon (which is less interesting).
-
Bonus/Optional:
Othello/Reversi
In a file named Hw6BonusOthello.java, write a
two-player game of Othello (also known as Reversi). If you do not know
how to play this game, the rules are simple and it is quick to learn (though
hard to master). You can learn about the game at the Wikipedia page:
http://en.wikipedia.org/wiki/Reversi
You can also play the game against a computer by searching for "Othello
Applet" in the search engine of your choice. (Of course, it is also
possible to find some Java source code for Othello by a similar search, but
that is most definitely not allowed! Write the code yourself, and do
not look at anyone else's implementation!!!)
Your program will not play against a person. Instead, it will support
two people playing each other, in the same manner as your Connect4 game.
Your program should not allow illegal moves, however. It should also
automatically pass if a player has no legal moves, and automatically end the
game when neither player has a legal move. Keep score and provide a
generally pleasing and easy-to-use UI. Have fun with it!
Carpe diem!