Computer Science 15-110 (Lecture 4), Spring 2010
Homework 6b
Due: Thu 25-Feb-2010 at 10pm (see hw6 for details)
This part of hw6 is non-collaborative (so you
must work alone).
The same rules as hw4 apply (read them carefully!).
- isFibby and nthFibby
- sumOfInverseOddSquares
- swirlImage
-
Bonus/Optional:
Better swirlImage
-
Bonus/Optional:
Ramanujan Inverse Pi
- isFibby and nthFibby
We will say that a positive integer n is fibby (an invented term)
if at least one of (5n2+4) or (5n2-4) is
a perfect square. So, for example:
* 1 is fibby because (5*12-4)
= 1 = 12. Also, though not required, (5*12+4)
= 9 = 32.
* 2 is fibby because (5*22-4)
= 16 = 42, even though (5*22+4)
= 24 is not a perfect square.
* 3 is fibby because (5*32+4)
= 49 = 72, even though (5*32-4)
= 41 is not a perfect square.
* 4 is not fibby because (5*42-4)
= 76 (not a perfect square) and (5*42+4)
= 84 (also not a perfect square)
Knowing this, write the methods isFibby and nthFibby. When you are
done, take a look at the first 10 or 15 fibby numbers, and see why we
invented this particular (silly, but not random) name. Cool!
- sumOfInverseOddSquares
The technique Euler used to solve the famous Basel Problem actually
found many other fascinating formulas relating to
π. For example, by only summing
the inverse odd squares, he obtained another expression involving
π2, only this time divided by
a different small positive integer d:
1/12 + 1/32 + 1/52 + ... =
π2/d (for some small positive
integer d)
For this problem, use Java (and not Google or a math textbook) to discover
the value of d in this formula, and then verify that the formula is true to
a very high accuracy if enough terms are used. Your solution should
include the code you used to discover the value of d, as well as the code to
verify it.
- swirlImage
Expanding on the rotateImage problem
in hw6a, an image can be swirled, rather than
merely rotated, if the angle of rotation θr
increases as the distance r increases from the origin. Using this
technique, write the swirlImage method that takes two parameters that are
similar to rotateImage -- an image and a double specifying an angle
θmax in degrees -- and returns a swirled image, where the
given angle θmax is the
largest angle of rotation of the swirl.
So, how do you find θr for
any source pixel (xs,ys)? First, before you loop
over each point, find dmax, the maximum distance from the center
to any pixel (for this value, we will use the distance from the image's
center to any of the image's corners, even if that corner would be mapped
outside of the target image).
Then, find a linear function θr(d)
so that there is no rotation at the center of the image and max rotation at
the farthest point. That is, find a linear function θr(d)
so that θr equals 0 (no
rotation) when d is 0 (at the center), and θr
equals θmax (max rotation)
when d is dmax (at the farthest point). Hint: this is
a simple linear function (a "direct variation", in fact). Now that you
have this function, you can loop over each pixel (xs,ys),
compute the distance d from the center of the image to the point (xs,ys),
and then use θr
(d) as the angle of rotation for that pixel, which finally is rotated just
as in the original rotateImage method.
Note: sure, this is a bit "mathy", but as is so often the case in
programming, a bit of algebra, geometry, trig, and sometimes even calculus
is often required when solving really interesting, compelling problems that
didn't seem mathy to begin with. For example, this problem is visual,
involving graphics and art. But the solution involves algebra and trig
(though not too much of either), not to mention logic. And that is why
we study those things, even if our target problem domains -- the ones you
are interested in -- are not themselves all that mathy.
-
Bonus/Optional:
Better swirlImage
As specified, the swirlImage method suffers a notable problem: many
target pixels will not be mapped to by source pixels, and so will be left
blank creating strange and unwanted artifacts throughout the target image.
For bonus, you can fix this in a method named betterSwirlImage. You
can do this any way you wish, but here is one way: rather than loop
over every source pixel, loop instead over every target pixel. Then,
basically run the math from above in reverse, so that you find the
corresponding source pixel location. But find this value as an ordered
pair of doubles, not ints. This "virtual pixel" will therefore sit
between 4 actual pixels that use integer coordinates (see?). So use
the weighted average of these 4 real pixels, based upon their
relative distances to the virtual pixel.
-
Bonus/Optional:
Ramanujan Inverse Pi
The great mathematician Ramanujan discovered many amazing formulas.
Here is one:
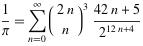
Write a method, ramanujanInversePi, that verifies this formula is true to a
high accuracy if enough terms are used. Hint: to avoid overflow,
it may help to make n a double value, even though it is an integer in the
formula.
Carpe diem!